Formatting the code in your patch for LibreOffice
Do you want to submit a patch to LibreOffice Gerrit, and you’re wondering if your code will be accepted or not? Other than providing a good solution to resolve a problem (fix a bug, implement a feature or enhancement), you should care about the code conventions, and in particular, code formatting. Suitable code formatting for LibreOffice is what we discuss here.
Code Formatting Conventions
LibreOffice is based on the old code base of OpenOffice. Because of that, LibreOffice uses the coding conventions of OpenOffice in most places. There is a list of rules that you can refer to when needed, in the OpenOffice Wiki:
Here is a comprehensive list of C++ coding rules:
You can see many rules and standards there – beyond code formatting – from design to the way you should declare and implement classes, and their functions and variables. You will also find topics about how to create header files, how to document code and how to achieve type safety.
But, focusing only on C++ code formatting, you should make sure that:
- Each source file should have a suitable header. For the existing files, please keep the header as is.
- The source file should have a newline character in the end.
- It is suggested that you limit the code to only ASCII characters, and avoid using utf-8 string literals directly.
- Indent your code with 4 spaces
- You should avoid tabs.
These rules have their own name in the OpenOffice code formatting conventions.
Let’s look at this code snippet from a C++ file: chart2/qa/extras/charttest.hxx
.
uno::Sequence < OUString > ChartTest::getImpressChartColumnDescriptions( std::u16string_view pDir, const char* pName ) { uno::Reference< chart::XChartDocument > xChartDoc = getChartDocFromImpress( pDir, pName ); uno::Reference< chart::XChartDataArray > xChartData ( xChartDoc->getData(), uno::UNO_QUERY_THROW); uno::Sequence < OUString > seriesList = xChartData->getColumnDescriptions(); return seriesList; }
As you can see, in the old OpenOffice style, there is a space after the starting parenthesis (, and also before the ending parenthesis ). Also, before and after <>. This might look old fashioned, but this is the way old code is written, and you are supposed to avoid changing the old code only for making it look better! I am going to describe why.
Why Keep Old Style Formatting?
There is a very good reason to keep the old style code formatting. To understand the code, and possible problems, developers usually refer to the code, and its history. Thus, it is important to keep the history of the code clean.
To achieve this, experienced developers suggest that you should avoid changing the formatting of the code, even if you are tempted to do so! Changing spacing, curly braces and other things in order to look nicer will pollute the git history.
The nice tool git blame
can show who to blame for each line of code! If you change the code format randomly, you may prevent other developers to understand the root cause of the change, and thus fixing the problems more difficult.
What to Do With the New Files?
For the newer files, the story is different! You are not only encouraged to use the nice looking new coding style, but git hooks also prevents you from submitting a code that does not conform with the new code formatting conventions! If your code does not conform to the new code conventions, you will see this error message:
ERROR: The above differences were found between the code to commit
and the clang-format rules. Tips:
- You may run '/opt/lo/bin/clang-format -i <problematic file>' to fix up style automatically.
- See solenv/clang-format/README on where to get the required version of clang-format binaries.
- If you renamed an excluded file, update solenv/clang-format/excludelist accordingly to keep it excluded.
solenv/clang-format/check-last-commit: KO
Makefile:485: recipe for target 'clang-format-check' failed
make: *** [clang-format-check] Error 1
As the tip suggests, there is a tool named clang-format
that does the formatting automatically for you. You only need to invoke:
/opt/lo/bin/clang-format -i <your-new-source-file.cxx>
You can read more about how to set up and use clang-format
here:
One nice thing about thing about clang-format
binary is that you can instruct your IDE to use this binary to do the formatting for you with a simple shortcut.
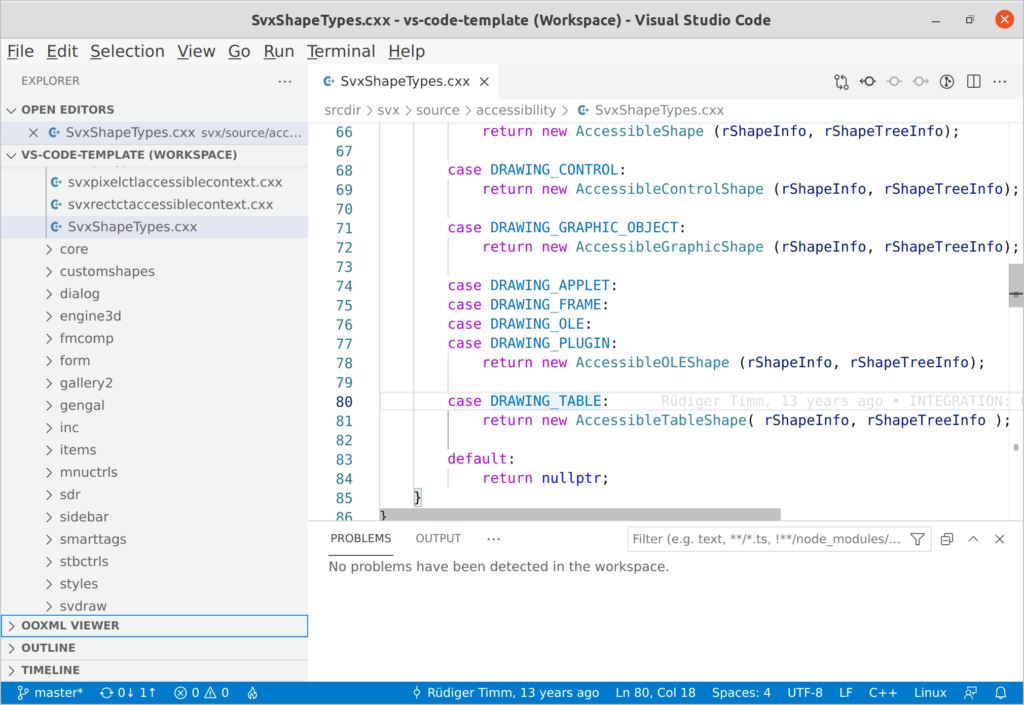
LibreOffice source code in Visual Studio Code
Each new file should have appropriate header and footer. You can look at TEMPLATE.SOURCECODE.HEADER and find an appropriate header and footer for you source code.
Final Notes
If you had problems with code formatting, try to read the above instructions and clean up your code. If you have tried but you couldn’t, that’s not a problem! Just ask me for help. I will try to help you format your code according to the LibreOffice coding conventions. Be bold and try to have your code merged in the LibreOffice code! You can learn more about how to get started with LibreOffice development here: